Investigating a single neuron
In this task you can familiarise yourself with the way you will interact with the BrainScaleS-2 chip during the lab exercises. For all tasks there exist jupyter notebooks that are intended to be used as a basis for the results. If you are not familiar with python or jupyter notebooks before beginning this lab course, we recommend that you spend some time beforehand to familiarise yourself.
During the execution of some of the notebook cells, you will cause an experiment to be run on a BrainScaleS-2 system remotely. You will find that due to the analog nature of some of the system’s implementation the execution is not deterministic, i.e., not each hardware execution will result in the same outcome.
Generally speaking the simplest hardware experiment can be divided in three steps
hardware configuration
hardware execution
analysis of hardware observables.
More sophisticated experiments can involve multiple iterations of these basic three steps or a subset of them. At the level of abstraction you will be working on for these lab exercises, most of the intricacies of the hardware configuration and execution will be hidden behind a common high-level API, called PyNN.
In the next section we will set up a connection to an RPC server that multiplexes connections to the hardware, which will allow us to work with the system interactively. This process is handled by a custom microscheduler (quiggeldy), a conceptual view of which you can see in the following figure. The actual hardware execution time has been colored in blue.
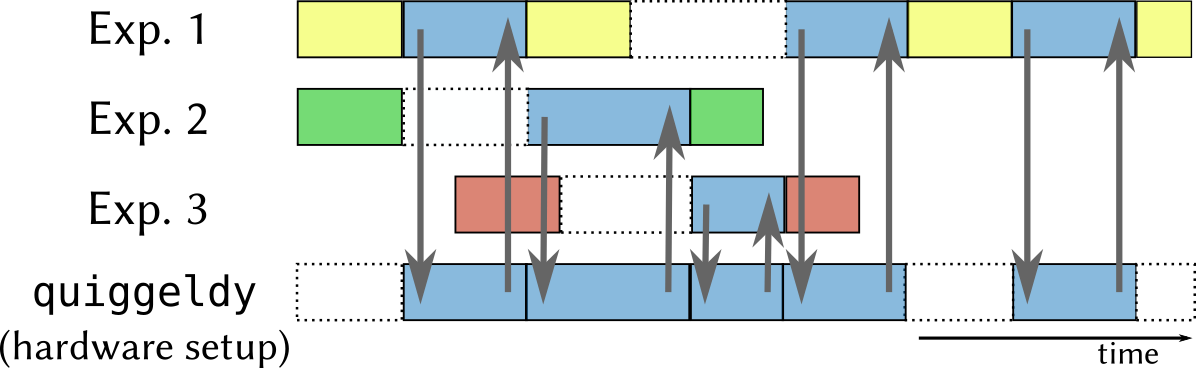
At the end of this notebook you will find a number of exercises to complete.
Experiment setup
Note
This print-friendly version of the instructions only lists the most central functions and code blocks. The interactive variant provides additional helpers.
For our first experiment, we create a single neuron and record its spikes as well as its membrane potential.
def experiment(title, v_leak, v_threshold, v_reset, i_bias_leak, savefig = False):
"""
Set up a leak over threshold neuron.
:param v_leak: Leak potential.
:param v_threshold: Spike threshold potential.
:param v_reset: Reset potential.
:param i_bias_leak: Controls the leak conductance (membrane time constant).
:param savefig: Save the experiment figure.
"""
plt.figure()
plt.title(title)
# everything between pynn.setup() and pynn.end()
# below is part of one hardware run.
pynn.setup()
# a pynn.Population corresponds to a certain number of
# neuron circuits on the chip
pop = pynn.Population(1, pynn.cells.HXNeuron(
# Leak potential, range: 400-1000
leak_v_leak=v_leak,
# Leak conductance, range: 0-1022
leak_i_bias=i_bias_leak,
# Threshold potential, range: 0-500
threshold_v_threshold=v_threshold,
# Reset potential, range: 300-1000
reset_v_reset=v_reset,
# Membrane capacitance, range: 0-63
membrane_capacitance_capacitance=63,
# Refractory time (counter), range: 0-255
refractory_period_refractory_time=255,
# Enable reset on threshold crossing
threshold_enable=True,
# Reset conductance, range: 0-1022
reset_i_bias=1022,
# Increase reset conductance
reset_enable_multiplication=True))
pop.record(["v", "spikes"])
# this triggers a hardware execution
# with a duration of 0.2 ms
pynn.run(0.2)
plot_membrane_dynamics(pop, ylim=(100, 800))
if savefig:
plt.savefig(results_folder.joinpath(f'fp_task1_{time.strftime("%Y%m%d-%H%M%S")}.png'))
plt.show()
mem = pop.get_data("v").segments[-1].irregularlysampledsignals[0]
spikes = pop.get_data("spikes").segments[-1].spiketrains[0]
pynn.end()
return mem, spikes
Exercises
A continuously spiking neuron
As a first exercise, set the neuron up such that it spikes continuously without any external input. For that purpose, play with the function’s parameters and note down a suitable configuration in the following cell. Make sure to also save the resulting plot.
mem, spikes = experiment(
title="A continuously spiking neuron",
v_leak = 1000,
v_threshold = 500,
v_reset = 400,
i_bias_leak = 150,
savefig = True
)
Simple spike train statistics
Calculate the neuron’s average firing rate.
Derive the inter-spike intervals (ISIs) of the spike train recorded in the previous exercise. The inter-spike interval denotes the time between two consecutive spikes of a neuron. Plot a histogram of the ISIs.
Identify a method to calculate the mean and standard deviation of the neuron’s instantaneous firing rate. The instantaneous firing rate provides a moment-by-moment measure of the neuron’s activity.
Hint: You may use, e.g., np.diff, np.mean, and np.std to complete these tasks.
Recording the f-I curve of a silicon neuron
Next, we want to study the behavior of a single neuron stimulated with a current source. For this, we introduce a new parameter for our neuron model. This will allow to enable/disable a constant current source and observe the impact.
Add constant_current_enable (True/False) and constant_current_i_offset (range=0-1022) as parameters into your experiment
Configure a non firing neuron
Vary the current and observe the impact
interact(
experiment,
title="Task 2: Current introduced firing",
v_leak=IntSlider(min=400, max=1022, step=1, value=1000),
v_threshold=IntSlider(min=0, max=500, step=1, value=500),
v_reset=IntSlider(min=300, max=1022, step=1, value=400),
i_bias_leak=IntSlider(min=0, max=1022, step=1, value=150),
current=IntSlider(min=0, max=1022, step=1, value=300),
)
To quantify your observations write a program that sweeps over a current range from 0 up to 800.
Plot the neuron’s mean instantaneous firing rate including its error over current.
Synaptic stimuli and PSP stacking
In the previous tasks, we analyzed the spiking dynamics of an isolated neuron. In this task, we want to look at the membrane response of the neuron to stimulations of incoming spikes. Your goal is to reproduce the image of PSP-Stacking (summation) from the introduction. For this, you might find it helpful to look into pynn_introduction again.
A remark about the calibration loaded above:
While this calibration is not needed for the simple experiments above, it is essential when looking at synaptic inputs.
Therefore, you should load it when setting up this experiment using: pynn.setup(initial_config=calib)
.
Steps:
Set up a population of one neuron with the default neuron parameters to record its membrane potential.
Create a population of one SpikeSourceArray as a stimulating population.
Connect the stimulating population to the neuron population.
Find a suitable spike pattern to recreate the plot (you might require multiple spikes).
Can the neuron spike with the current neuron configuration? Explain.
Suggest and implement changes in the configuration so that the neuron spikes. Try to apply one change at a time. Explain your choices.
Can you tell which changes can be theoretically equivalent?
Hint: Changes can be related to neuron parameters, projection, or population.